Table of Contents
Introduction to Go Programming Language
Welcome, aspiring programmers! Have you heard the buzz about Go, the versatile language taking the tech world by storm? Buckle up, because this comprehensive guide will equip you with everything you need to embark on your Go programming language adventure.
What is Go?
Go, also known as GoLang, is a powerful open-source programming language created by Google. It’s designed for building efficient and scalable applications, particularly for the cloud. Go boasts a clean, concise syntax, making it a breeze to learn and use, even for beginners.
Key Features and Benefits of Go:
- Simplicity: Go’s syntax is clear and easy to read, allowing you to focus on the logic of your program rather than complex language constructs.
- Concurrency: Go excels at handling concurrent tasks, making it ideal for building modern, responsive applications.
- Statically Typed: Go ensures type safety, preventing runtime errors and enhancing code reliability.
- Built-in Tools: Go comes with a rich set of tools for building, testing, and debugging your programs, streamlining the development process.
- Standard Library: Go offers a robust standard library, providing you with essential functionalities for common tasks.
Why Learn Go Programming Language?
Go is becoming extremely popular. Find out why you should think about including Go in your programming skills.
- Growing Popularity and Use Cases: Major companies like Google, Uber, Netflix, and Dropbox rely on Go to power their infrastructure. Go’s demand in the job market is high, making it a valuable skill for career advancement.
- Advantages over Other Programming Languages: Compared to languages like Java or C++, Go offers faster compilation times, simpler syntax, and built-in concurrency features.
Installing Go Programming Language
Before jumping into coding, let’s get your development environment set up. Here’s how to install the Go compiler:
Downloading the Go Compiler:
Visit the Go website at https://go.dev/doc/install and get the installer that matches your operating system (Windows, macOS, or Linux).
Setting Up Your Development Environment:
Once downloaded, follow the installation instructions. This will typically involve setting up your PATH environment variable to point to the Go installation directory. This allows you to run Go commands from your terminal.
Integrating Go with Your Preferred Code Editor:
Many popular code editors, like Visual Studio Code or GoLand, offer built-in Go support. This provides features like syntax highlighting, code completion, and debugging tools, making your coding experience more efficient.
Writing Your First Go Program
Now, let’s get your hands dirty with some Go code!
Creating a New Go File:
Open your preferred code editor and create a new file with the extension .go. We’ll call this file hello.go.
Printing “Hello, World!”
Here’s a simple Go program that prints the classic “Hello, World!” message:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Understanding Go Syntax:
Let’s break down this program:
package main
: This line specifies the package name, which ismain
for standalone programs.import "fmt"
: This line imports thefmt
package, which provides functions for formatted printing.func main() { ... }
: This defines themain
function, which is the entry point of your program.fmt.Println("Hello, World!")
: This line calls thePrintln
function from thefmt
package to print the message “Hello, World!” to the console.
Go Programming Language Fundamentals
Now that you’ve tasted the sweetness of the Go programming language, let’s delve deeper into some essential Go concepts.
Data Types and Variables:
- Primitive Data Types: Go offers basic data types like
int
(integers),float
(floating-point numbers),bool
(Booleans – true or false), andstring
(text). - Declaring and Initializing Variables: You declare variables using the
var
keyword followed by the variable name and data type. You can optionally assign an initial value during declaration. - Type Conversion and Type Inference: Go allows you to convert variables between compatible data types. It can also infer the type of a variable based on the assigned value.
Control Flow
Making decisions and repeating actions are fundamental aspects of programming. Go provides various control flow constructs to manage the flow of your program’s execution.
- If-else Statements: The
if-else
statement allows you to execute code conditionally based on a boolean expression.
age := 25
if age >= 18 {
fmt.Println("You are eligible to vote.")
} else {
fmt.Println("You are not eligible to vote yet.")
}
- Switch Statements: The
switch
statement is used for multi-way branching based on the value of a variable.
day := "Monday"
switch day {
case "Saturday", "Sunday":
fmt.Println("It's the weekend!")
case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday":
fmt.Println("It's a weekday. Hustle hard!")
default:
fmt.Println("Invalid day!")
}
- Loops (for loop): Loops allow you to execute a block of code repeatedly until a certain condition is met. The for loop is a versatile looping construct in Go.
for i := 1; i <= 5; i++ {
fmt.Println("Iteration", i)
}
Functions
Functions are code blocks that can be used over and over to do certain jobs. They promote code modularity and maintainability.
- Defining and Calling Functions:
func greet(name string) {
fmt.Println("Hello,", name)
}
func main() {
greet("Go Programmer")
}
In this example, the greet
function takes a string argument (name
) and prints a greeting message. The main
function calls the greet
function, passing the desired name as an argument.
- Parameters and Return Values: Functions can take in parameters for input and give back return values. Parameters are used to send data to the function, while return values are used to send data back to the caller.
- Anonymous Functions and Closures: Go programming language supports anonymous functions, which are functions defined without a name. They are often used as callbacks or for short, inline functionality. Closures are anonymous functions that can access and retain variables from their enclosing scope, even after the function has returned.
Related Article: A Guide to Top 20 Coding Languages List
Arrays, Slices, and Maps
Go provides various data structures to organize your data efficiently.
- Declaring and Using Arrays: Arrays are fixed-size collections of elements of the same data type.
var numbers [5]int
numbers[0] = 10
numbers[1] = 20
fmt.Println(numbers) // Output: [10 20 0 0 0]
- Slices: Dynamic Arrays: Slices are dynamic arrays that can grow or shrink in size as needed. They offer a more flexible alternative to fixed-size arrays.
fruits := []string{"apple", "banana", "orange"}
fruits = append(fruits, "mango") // Add element
fmt.Println(fruits) // Output: [apple banana orange mango]
- Maps: Key-Value Data Structures: Maps are unordered collections of key-value pairs. You can use any data type as keys and values.
customer := map[string]string{
"name": "Alice",
"email": "alice@example.com",
}
fmt.Println(customer["name"]) // Output: Alice
Packages and Imports
Go promotes code organization with packages. Packages group related functionalities together.
- Organizing Code with Packages: Your Go code can be organized into packages. Each package resides in its directory. The file name within the directory should match the package name.
- Importing External Packages: Go allows you to import functionalities from external packages. The
import
statement is used to include packages in your code. - Creating and Using Your Packages: You can create your reusable packages by grouping related code and functions into a directory structure.
Next Steps
You’ve successfully grasped the fundamentals of the Go programming language! Now it’s time to expand your knowledge and explore more advanced concepts:
Exploring More Advanced Go Features:
- Concurrency Channels: Go excels at handling concurrent tasks. Channels provide a powerful mechanism for communication and synchronization between concurrent Go routines (lightweight threads). Mastering channels allows you to build highly scalable and responsive applications.
- Interfaces: A type must implement a set of methods defined by interfaces. They promote code flexibility and decoupling, making your code more maintainable and reusable.
- Pointers: Pointers are variables that store memory addresses. Understanding pointers unlocks advanced Go features like memory manipulation and efficient data structures. However, pointers can be tricky for beginners, so proceed with caution and practice!
- Error Handling: Robust error handling is crucial for writing reliable Go applications. Go programming language provides built-in mechanisms for handling errors gracefully, ensuring your programs function predictably even in unexpected situations.
Building Simple Projects to Practice Go:
Practicing is the most effective method to strengthen your understanding. Here are some ideas for beginner-friendly Go projects:
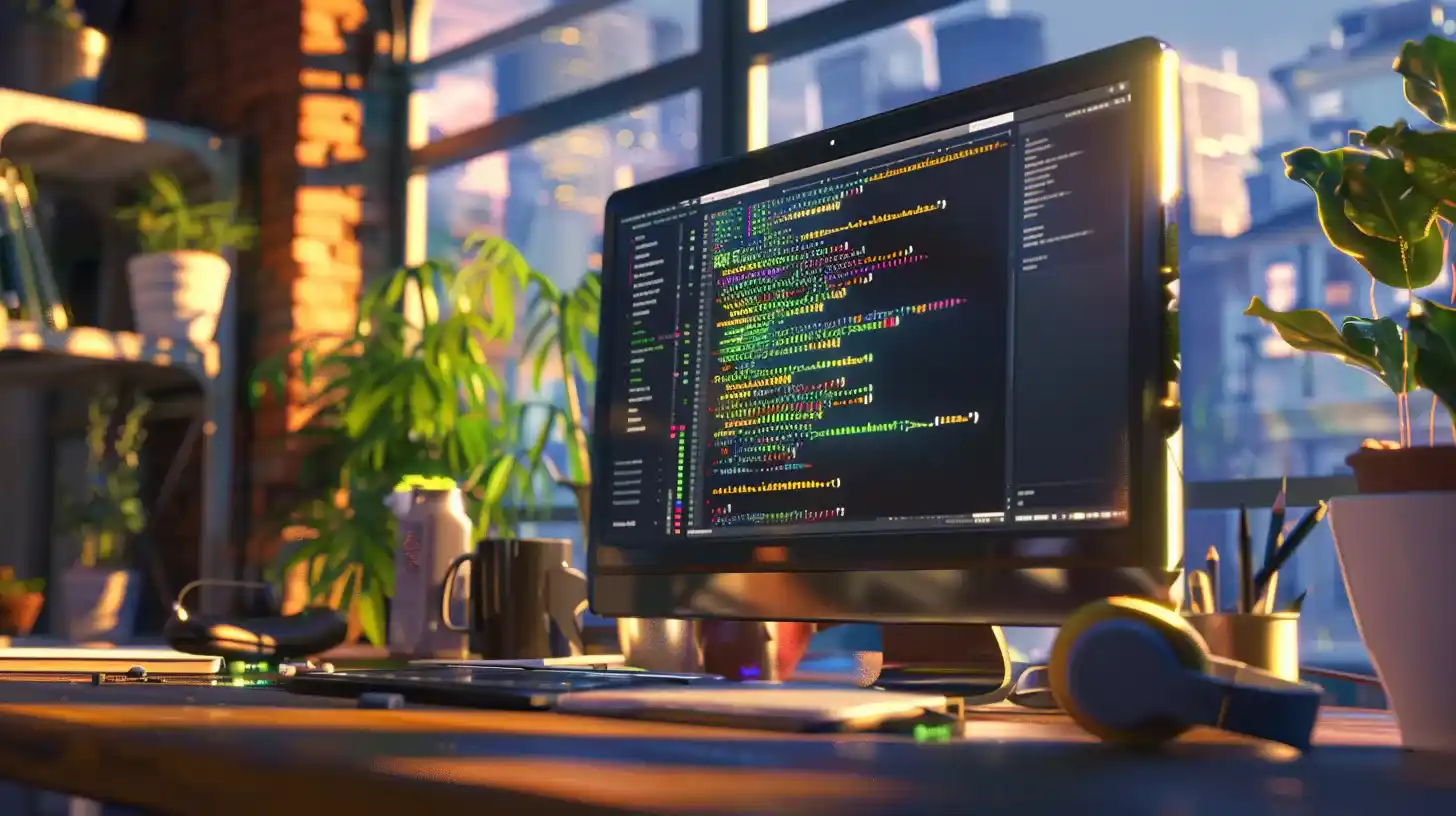
- Command-line Tools: Build a simple command-line calculator, currency converter, or text manipulation tool. These projects help you practice basic functionalities and work with user input.
- Web Server: Create a basic web server using frameworks like Echo or Gin. This introduces you to web development concepts in Go programming language and prepares you for building more complex web applications.
- Data Processing Tools: Write a program that reads data from a file, performs calculations, and generates output. This helps you practice working with files and manipulating data in Go.
Here are some project ideas to help you begin. Don’t be afraid to experiment and explore your interests! As you progress, consider contributing to open-source projects to gain real-world experience and collaborate with other Go programming language developers.
Related Article: AI Coding Tools: Unlock Future Success with 10 Innovations
Resources for Further Learning and Growth:
The Go official website (https://go.dev/) offers extensive resources to support your Go programming language journey:
- Documentation: The official documentation provides comprehensive explanations of Go syntax, features, standard library packages, and best practices.
- Tutorials: Step-by-step tutorials guide you through various Go programming language concepts, from basic syntax to building web applications.
- Blog: The Go blog features articles, announcements, and insights from experienced Go developers, keeping you updated on the latest advancements.
- Play.golang.org: This interactive playground allows you to experiment with Go code snippets directly in your browser, making it a convenient tool for learning and testing code.
Beyond the official resources, numerous online courses, tutorials, and communities cater to Go developers. Explore these resources to find learning methods that suit your style and pace.
Join the Go Community:
The Go community is welcoming and supportive. Here are ways to connect with other Go developers:
- Go Forums: The official Go forums (https://forum.golangbridge.org/) are a great platform to ask questions, share your code, and learn from other developers.
- Stack Overflow: Stack Overflow (https://stackoverflow.com/questions/tagged/go) is a vast repository of questions and answers related to the Go programming language. Search for solutions or post your questions to get help from the wider developer community.
- Meetup Groups: Search for local Go meetup groups in your area to connect with fellow Go enthusiasts and participate in workshops or discussions.
By actively engaging with the Go community, you can accelerate your learning, stay updated on the latest developments, and build valuable connections within the Go ecosystem.
Conclusion
This guide has equipped you with a solid foundation for your Go programming language adventure. Remember, the key to mastering Go is continuous learning and practice. Embrace the journey, explore new challenges, and don’t hesitate to seek help from the vibrant Go community. With dedication and perseverance, you’ll be well on your way to becoming a proficient Go programmer!