Table of Contents
PostgreSQL stands as one of the most powerful open-source relational database management systems available today. Its rich feature set includes numerous built-in functions that can dramatically simplify complex data operations. In this comprehensive guide, we’ll explore the 20 most essential PostgreSQL functions that every developer should have in their toolkit, complete with practical examples and real-world applications.
Understanding PostgreSQL Functions
Before diving into specific functions, it’s important to understand that PostgreSQL functions can be categorized into several types:
- Aggregate functions that operate on sets of rows
- Window functions for analytical operations
- Scalar functions that operate on single values
- Table functions that return multiple rows
- String manipulation functions
- Date/Time functions
Let’s explore the most useful functions in each category.
1. Text Manipulation with string_agg()
String aggregation is a common requirement in database operations, particularly when generating reports or preparing data for display. The string_agg() function concatenates row values into a single string, making it invaluable for creating comma-separated lists or combining text data.
SELECT department, string_agg(employee_name, ', ' ORDER BY employee_name)
FROM employees
GROUP BY department;
This function is particularly powerful because it:
- Allows ordering of elements before aggregation
- Supports custom separators
- Can handle NULL values gracefully
- Works with GROUP BY operations efficiently
Real-world application: Generating report summaries where multiple items need to be combined into a single cell, such as listing all skills for each employee.
2. Date Handling with date_trunc()
Time-series analysis often requires grouping data by specific time intervals. date_trunc() simplifies this process by allowing you to truncate timestamps to any desired precision level.
SELECT date_trunc('month', created_at) as month,
COUNT(*) as monthly_orders,
SUM(amount) as monthly_revenue
FROM orders
GROUP BY date_trunc('month', created_at)
ORDER BY month;
Common use cases include:
- Monthly/quarterly/yearly reporting
- Time-based data aggregation
- Financial period analysis
- User activity tracking
3. Dynamic Series Generation with generate_series()
The generate_series() function creates sequential data, which is particularly useful for filling gaps in time series data or creating test data.
-- Generate dates for an entire year
SELECT generate_series(
'2024-01-01'::date,
'2024-12-31'::date,
'1 month'::interval
) as dates;
-- Generate numerical sequences with custom steps
SELECT generate_series(1, 100, 5) as number_sequence;
This function excels in:
- Creating date ranges for reporting
- Generating test data
- Filling gaps in sparse data sets
- Creating regular intervals for analysis
4. JSON Manipulation with jsonb_set()
As JSON data becomes increasingly common in modern applications, jsonb_set() provides a clean way to modify JSON documents without replacing them entirely. This function is particularly important in today’s microservices architectures where JSON is the de facto standard for data exchange.
-- Update nested JSON values
SELECT jsonb_set('{"user": {"name": "John", "age": 30}}'::jsonb,
'{user,age}',
'35');
-- Add new fields to existing JSON
SELECT jsonb_set(
data::jsonb,
'{preferences}',
'{"theme": "dark", "notifications": true}'::jsonb
)
FROM user_profiles;
Key benefits include:
- Atomic updates to JSON fields
- Preservation of existing data
- Support for nested structures
- Type safety
5. Array Operations with array_agg()
The array_agg() function aggregates values into an array, perfect for collecting related items into a single field. This is particularly useful when dealing with one-to-many relationships or when you need to process multiple related values together.
-- Basic array aggregation
SELECT category_id,
array_agg(DISTINCT tag_name ORDER BY tag_name) as tags,
array_agg(product_id) as product_ids
FROM product_tags
GROUP BY category_id;
-- Combining with other aggregates
SELECT department,
array_agg(employee_name) as employees,
COUNT(*) as employee_count,
AVG(salary) as avg_salary
FROM employees
GROUP BY department;
Common applications include:
- Tag management systems
- Feature lists for products
- Related item grouping
- Multi-value attribute storage
6. Full-Text Search Capabilities
PostgreSQL’s full-text search functions provide powerful text search capabilities that go beyond simple LIKE queries. The combination of to_tsvector() and to_tsquery() enables efficient text search operations with language-aware features.
-- Create a search index
CREATE INDEX idx_fts_content ON documents
USING gin(to_tsvector('english', content));
-- Perform full-text search
SELECT title,
ts_rank(to_tsvector('english', content),
to_tsquery('english', 'postgresql & database')) as rank
FROM documents
WHERE to_tsvector('english', content) @@ to_tsquery('english', 'postgresql & database')
ORDER BY rank DESC;
Key features include:
- Language-aware stemming
- Ranking of results
- Support for complex search expressions
- High performance with appropriate indexing
7. Window Functions for Analysis
Window functions enable sophisticated data analysis by performing calculations across sets of rows while still returning detail-level data.
SELECT
product_name,
category,
price,
avg(price) OVER (PARTITION BY category) as avg_category_price,
price - avg(price) OVER (PARTITION BY category) as price_diff_from_avg,
rank() OVER (PARTITION BY category ORDER BY price DESC) as price_rank
FROM products;
Applications include:
- Competitive analysis
- Performance rankings
- Running totals
- Moving averages
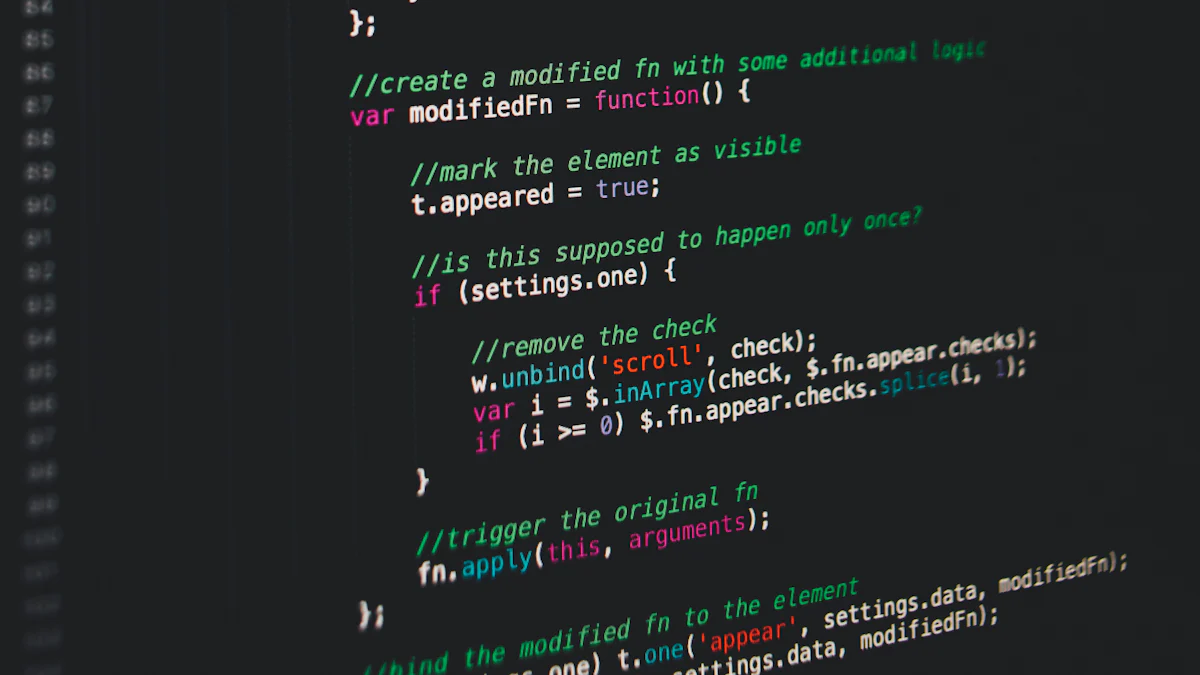
8. Null Value Handling with coalesce()
The coalesce() function provides elegant null value handling by returning the first non-null value in a list. This is essential for data cleaning and report generation.
SELECT
product_name,
coalesce(discount_price, regular_price) as final_price,
coalesce(description, 'No description available') as product_description,
coalesce(category, 'Uncategorized') as product_category
FROM products;
Common applications include:
- Default value handling
- Report generation
- Data cleaning
- API response formatting
9. Date Component Extraction with extract()
The extract() function is essential for temporal analysis, allowing you to pull specific components from date/time values.
SELECT
extract(YEAR FROM order_date) as year,
extract(MONTH FROM order_date) as month,
extract(DOW FROM order_date) as day_of_week,
sum(amount) as total_sales
FROM orders
GROUP BY 1, 2, 3
ORDER BY 1, 2, 3;
Key use cases include:
- Sales analysis by time period
- Seasonal trend analysis
- Day-of-week patterns
- Custom calendar calculations
10. Regular Expression Operations with regexp_replace()
PostgreSQL’s regexp_replace() function enables powerful text manipulation using regular expressions.
SELECT
regexp_replace(phone_number,
'(\d{3})(\d{3})(\d{4})',
'(\1) \2-\3') as formatted_phone,
regexp_replace(email, '@.*$', '@[hidden]') as masked_email
FROM contacts;
Common uses:
- Data formatting
- Text cleaning
- Pattern matching
- Data masking
11. Age Calculation with age()
The age() function calculates the precise interval between timestamps or dates.
SELECT
first_name,
birth_date,
age(birth_date) as current_age,
age(hire_date, birth_date) as hire_age
FROM employees;
Applications include:
- Employee analytics
- Customer demographics
- Service duration calculations
- Age-based segmentation
12. Statistical Analysis with percentile_cont()
This function calculates continuous percentiles, perfect for statistical analysis.
SELECT department,
percentile_cont(0.5) WITHIN GROUP (ORDER BY salary) as median_salary,
percentile_cont(ARRAY[0.25, 0.5, 0.75])
WITHIN GROUP (ORDER BY salary) as salary_quartiles
FROM employees
GROUP BY department;
13. Lead and Lag Functions
These window functions access data from subsequent or previous rows.
SELECT
order_date,
amount,
lag(amount) OVER (ORDER BY order_date) as previous_amount,
lead(amount) OVER (ORDER BY order_date) as next_amount,
amount - lag(amount) OVER (ORDER BY order_date) as amount_change
FROM orders;
14. Array Element Operations with unnest()
The unnest() function converts array elements into rows, enabling detailed analysis of array data.
SELECT unnest(tags) as individual_tag,
COUNT(*) as tag_count
FROM products
GROUP BY individual_tag;
15. Date Arithmetic with interval
While not strictly a function, interval arithmetic is crucial for date calculations.
SELECT
order_date,
order_date + interval '30 days' as due_date,
order_date - interval '1 year' as year_ago,
CASE
WHEN current_date - order_date > interval '30 days'
THEN 'Overdue'
ELSE 'Current'
END as status
FROM orders;
16. String Functions: split_part()
split_part() divides strings based on a delimiter and returns a specific segment.
SELECT
email,
split_part(email, '@', 1) as username,
split_part(email, '@', 2) as domain
FROM users;
17. Random Value Generation with random()
The random() function generates random values, useful for sampling and testing.
SELECT
id,
name,
random() as random_value
FROM products
ORDER BY random()
LIMIT 10;
18. Type Conversion with cast()
Type conversion is essential for data manipulation and compatibility.
SELECT
price::numeric(10,2) as formatted_price,
created_at::date as date_only,
amount::text as amount_string
FROM orders;
19. JSON Object Creation with json_build_object()
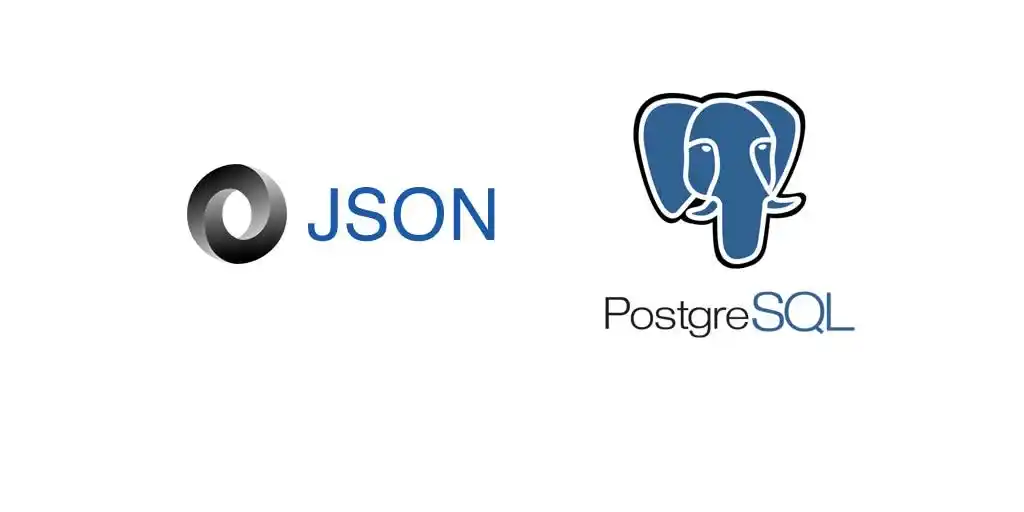
This function creates JSON objects from individual fields.
SELECT json_build_object(
'id', id,
'name', name,
'contacts', json_build_object(
'email', email,
'phone', phone
)
) as user_json
FROM users;
20. Text Search Configuration with to_tsquery()
This function configures full-text search queries with advanced options.
SELECT title
FROM documents
WHERE to_tsvector('english', content) @@
to_tsquery('english', 'postgresql & !mysql & (database | dbms)');
Best Practices and Considerations
When working with PostgreSQL functions, keep these important points in mind:
1. Performance Optimization
- Use appropriate indexes for function arguments
- Consider materialized views for expensive calculations
- Monitor query execution plans
- Cache frequently accessed results
2. Data Type Handling
- Choose appropriate data types for function parameters
- Handle NULL values explicitly
- Consider type conversion impacts
- Use appropriate casting methods
3. Error Handling
- Implement proper exception handling
- Validate input data
- Provide meaningful error messages
- Use transaction management appropriately
4. Maintenance and Documentation
- Document function usage and requirements
- Maintain version compatibility
- Regular performance monitoring
- Keep up with PostgreSQL updates
Future Trends in PostgreSQL Functions
As PostgreSQL continues to evolve, we’re seeing exciting developments in function capabilities:
- Enhanced JSON processing functions
- Machine learning integration
- Improved full-text search capabilities
- Better support for distributed computing
Conclusion
PostgreSQL’s rich set of built-in functions provides powerful tools for data manipulation and analysis. Understanding and effectively using these functions can significantly improve your database operations’ efficiency and maintainability. Regular practice with these functions will help you become more proficient in database development and optimization.
Remember that PostgreSQL is constantly evolving, with new functions and features being added in each release. Stay updated with the latest PostgreSQL documentation and community resources to make the most of these powerful database capabilities.
As you continue to work with PostgreSQL, experiment with combining different functions to create more sophisticated solutions. The real power of PostgreSQL often lies not in individual functions, but in how they can be combined to solve complex business problems efficiently and elegantly.