Table of Contents
Traditional coding education often focuses on writing programs from scratch, starting with syntax and gradually moving toward problem-solving. However, an alternative and highly effective approach is “Reverse Coding,” where learners improve their coding skills by debugging and analyzing existing software. This method not only enhances problem-solving abilities but also deepens understanding of code structure, logic, and best practices.
What is Reverse Coding?
Reverse Coding involves examining pre-written code, identifying issues, and fixing bugs to improve functionality. Instead of writing fresh code, learners work with existing applications, dissecting their logic and finding ways to optimize or enhance them. This approach is widely used in real-world software development, where developers spend a significant amount of time debugging rather than writing new code.
Why Learn Through Debugging?
- Develops Analytical Thinking – Debugging forces learners to think critically, analyze patterns, and understand the logic behind different coding constructs.
- Real-World Application – Most professional coding involves maintaining and improving existing code rather than writing everything from scratch.
- Enhances Problem-Solving Skills – By fixing errors, developers gain hands-on experience in troubleshooting, an essential skill in software development.
- Boosts Code Comprehension – Reading and understanding someone else’s code helps learners grasp different coding styles and best practices.
- Immediate Feedback Loop – Debugging provides instant results, allowing learners to see the impact of their changes in real time.
- Improves Efficiency – Understanding how existing code works helps in writing better and more efficient code in future projects.
- Encourages Collaboration – Working on debugging tasks often requires engaging with other developers, which improves communication and teamwork skills.
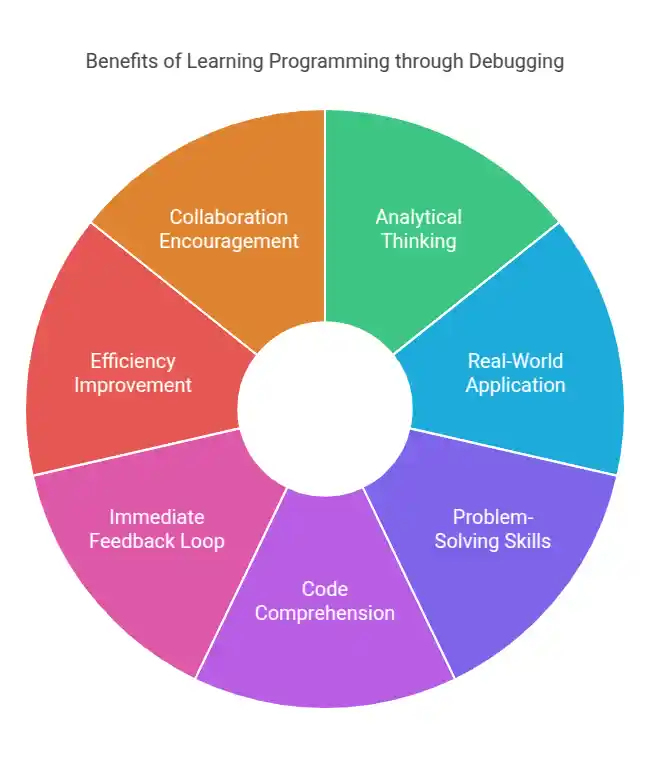
How to Get Started with Reverse Coding
- Pick an Open-Source Project – Websites like GitHub, GitLab, or Bitbucket offer a vast collection of open-source projects. Choose a project written in a language you are familiar with.
- Analyze the Code – Before fixing issues, spend time understanding the code structure, its purpose, and how different modules interact.
- Use Debugging Tools – Leverage debugging tools like breakpoints, logging, and unit tests to inspect the code and locate issues.
- Fix Bugs and Optimize Code – Start with simple bug fixes and gradually work on optimizing and improving performance.
- Compare Solutions – Look at how experienced developers solve similar issues by checking pull requests, discussions, and documentation.
- Contribute to Open-Source – Engaging with the developer community and contributing fixes to projects can boost confidence and learning.
- Experiment with Code Modifications – Try refactoring sections of code to see if they can be improved while maintaining functionality.
- Join Developer Forums – Platforms like Stack Overflow, Reddit, and Discord communities can help when encountering roadblocks.
- Work on Debugging Challenges – Participate in coding challenge platforms that offer debugging exercises to sharpen skills.
- Reverse Engineer Closed-Source Applications – Disassembling compiled code (where legally permitted) can provide deep insights into how software works.
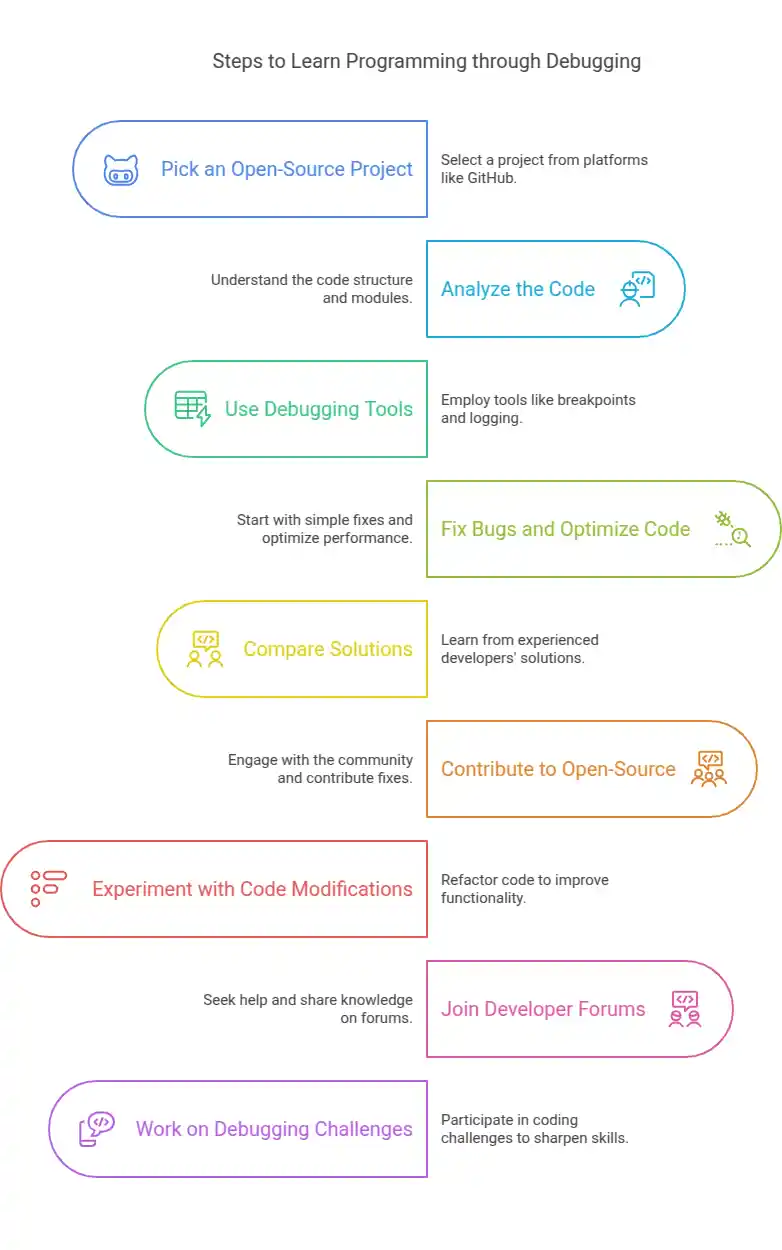
Advanced Benefits of Reverse Coding
- Strengthens Debugging Intuition – Over time, developers develop an instinct for identifying and fixing bugs quickly.
- Increases Adaptability – Understanding different codebases improves the ability to switch between various projects and technologies.
- Improves Documentation Writing Skills – Working with undocumented code encourages better commenting and documentation habits.
- Encourages Reverse Engineering Skills – Learning how software works under the hood can help with system design and cybersecurity.
- Teaches Efficient Code Structuring – By analyzing different architectures, learners can recognize the best ways to structure large-scale applications.
- Boosts Confidence in Working with Legacy Code – Many real-world applications involve working with outdated, legacy code that needs maintenance and upgrading.
- Develops Patience and Persistence – Debugging complex problems requires patience, which helps develop a problem-solving mindset beneficial in all areas of life.
- Enhances Code Review Skills – By analyzing and debugging code, developers gain experience in reviewing and providing constructive feedback on other programmers’ work.
- Refines Error Handling Techniques – Debugging teaches developers how to implement better error handling mechanisms, making software more robust and user-friendly.
- Improves Algorithmic Thinking – Understanding code logic through debugging helps in learning and optimizing algorithms, essential for high-performance applications.
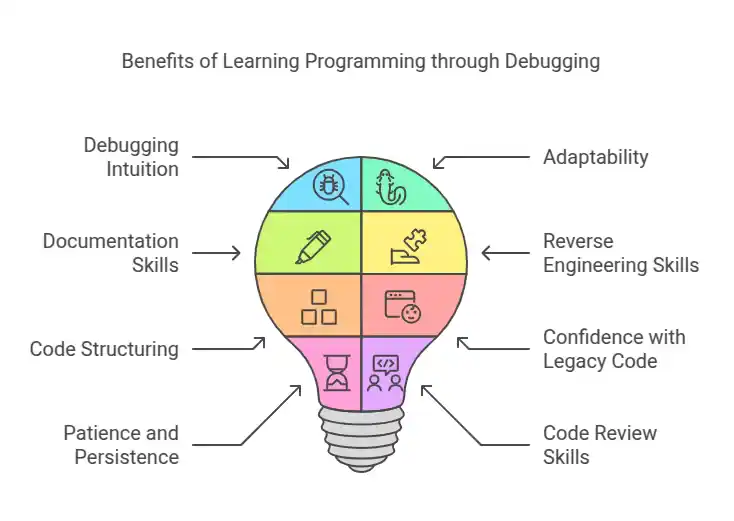
Common Challenges and How to Overcome Them
- Overwhelming Codebase – Large projects can be daunting. Start small by focusing on a specific module or bug.
- Understanding Complex Logic – Use tools like flowcharts or pseudocode to break down complex functions.
- Frustration with Errors – Debugging can be frustrating, but persistence and structured troubleshooting can ease the process.
- Lack of Documentation – If a project lacks documentation, explore forums, comments in the code, or ask the community for help.
- Dependency Conflicts – Some projects rely on outdated or incompatible dependencies; learning how to resolve these issues is a valuable skill.
- Code Readability Issues – Poorly written code can be hard to follow. Use tools like linters and formatters to improve readability.
- Difficulty in Debugging Third-Party Code – Some applications rely heavily on third-party libraries, making debugging difficult. Using proper logging and debugging tools can simplify the process.
- Time Management – Debugging can be time-consuming; setting goals and breaking tasks into smaller steps can help maintain productivity.
- Dealing with Recurring Bugs – Some bugs reappear in software due to hidden dependencies. Keeping a log of fixes and their effects can help prevent this issue.
- Understanding Code Refactoring – Debugging also involves improving code readability and maintainability. Learning when and how to refactor is crucial in making long-term improvements.
Real-World Applications of Reverse Coding
- Security Analysis – Ethical hackers and security analysts use reverse coding techniques to find vulnerabilities in software.
- Software Maintenance – Companies rely on reverse engineering to update and maintain legacy systems.
- Performance Optimization – Debugging helps identify bottlenecks and optimize code for better performance.
- Learning New Languages – Reverse coding is an effective way to learn new programming languages by studying existing implementations.
- Game Development – Game modders and developers analyze existing game code to modify or improve gameplay mechanics.
- AI and Machine Learning Debugging – Developers working with AI models often analyze pre-trained models and fine-tune them for better results.
- Mobile App Development – Reverse engineering existing mobile apps can help in understanding UI/UX patterns and improving performance.
- Embedded Systems Debugging – Engineers working with firmware or IoT devices frequently debug hardware-software interactions.
- Network Security Testing – Analyzing network traffic and debugging security flaws is a critical part of penetration testing.
- Automated Testing Development – Debugging automated test cases helps ensure continuous integration and delivery processes work correctly.
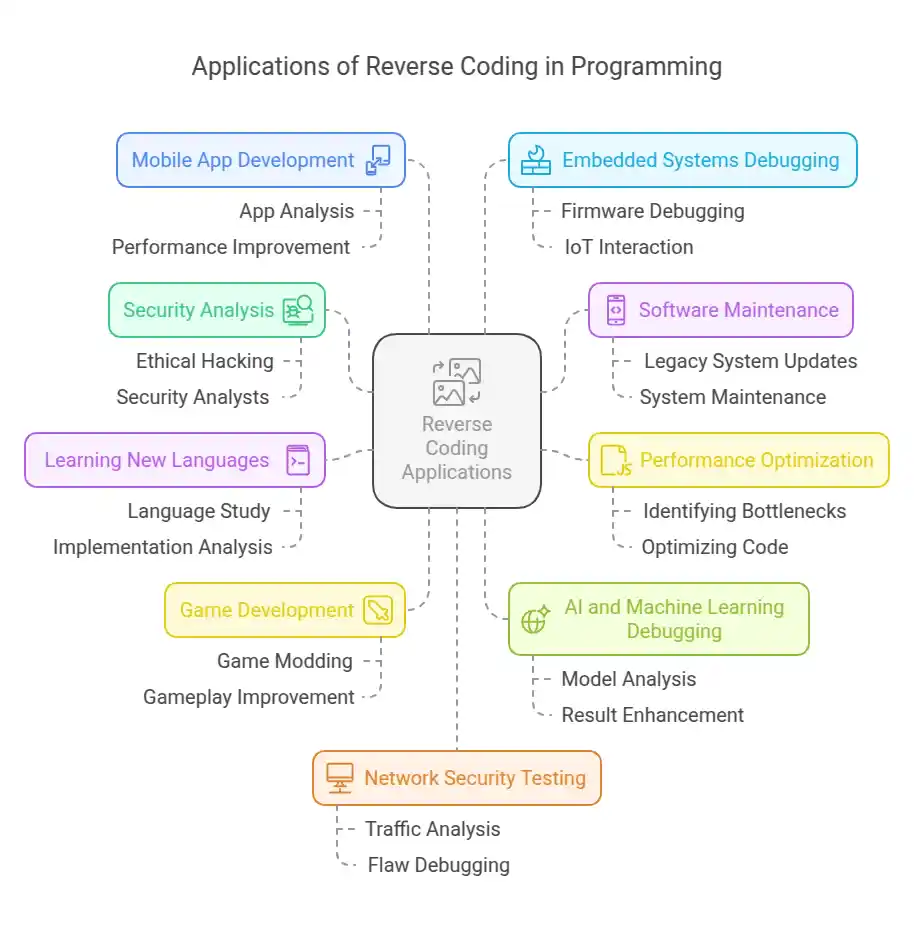
Techniques and Tools for Effective Reverse Coding
Mastering reverse coding means mastering the art of analyzing and understanding code. Here are some techniques and tools that can help you along the way:
- Static and Dynamic Analysis – Static analysis involves reviewing the code without executing it, allowing you to understand its structure and flow. Dynamic analysis, on the other hand, involves running the code to observe its behavior and output. Combining both techniques gives you a comprehensive view of how the code works.
- Version Control – Using version control systems (like Git) is invaluable when engaging with reverse coding. You can see the history of changes in a codebase, understand why certain decisions were made, and spot regressions or bugs that were introduced in later versions.
- Unit Testing – Implementing unit tests while debugging existing code can help you isolate specific bugs and track down issues faster. Writing tests for existing code also serves as a form of documentation, ensuring future developers can understand how the system behaves.
- Refactoring Tools – Tools like IDEs and code linters provide automatic suggestions for improving code readability and structure. Refactoring code in the process of debugging can also help improve the overall quality of the software.
- Interactive Debugging – Leverage interactive debugging tools to step through your code in real-time. Debuggers like GDB for C/C++ or built-in debuggers in modern IDEs (e.g., Visual Studio Code or PyCharm) allow you to pause, inspect variables, and understand how data is being manipulated line by line.
Reverse Coding as a Pathway to Mastery
At the core of reverse coding is the idea of continual growth through real-time feedback and engagement. When you debug and refactor code, you’re not just solving individual problems—you’re honing an essential skill that enables mastery over time.
- Learning by Doing – The hands-on nature of debugging accelerates the learning process, ensuring that concepts stick better than when learned in a theoretical environment. Reverse coding forces you to interact directly with the code, making it more intuitive and ingraining programming concepts in your memory.
- Building Strong Foundations – Debugging reveals gaps in knowledge, whether it’s a misunderstanding of a concept or a lack of familiarity with a tool. By addressing these gaps, you build stronger foundational skills that make future challenges easier to tackle.
- Building a Portfolio – Many developers build portfolios of open-source contributions, but a portfolio of well-documented, debugged, and optimized code showcases not only your ability to create but your skill in improving existing systems. This is a testament to your problem-solving mindset and deep technical understanding.
Conclusion
Reverse coding is more than just a technique for debugging or problem-solving—it’s a powerful tool for developers to engage with the code in an immersive and meaningful way. By practicing reverse coding, you gain valuable insights that can enhance your ability to think critically, optimize existing systems, and maintain a strong grasp on coding best practices.
Start small, dive deep into open-source projects, and let debugging reveal a whole new world of coding knowledge. Whether you’re looking to level up your skills for professional development, sharpen your problem-solving abilities, or even contribute to the community, reverse coding will lead you to mastery one bug at a time.
READ MORE:
Mastering Mobile Responsiveness: Building Websites That Stunning on Any Device
Effective AI in Hospitals: Reducing Burnout and Maximizing Efficiency